I have seen very common requirement to process the value selected in the Single Selection Combo Box at run time. Following example tells how to get this value at runtime in the backing bean for static list and dynamic list.
1. Static List
Static list allows to add a fixed list of user defined values. The following image shows the static list created on the Department Id.
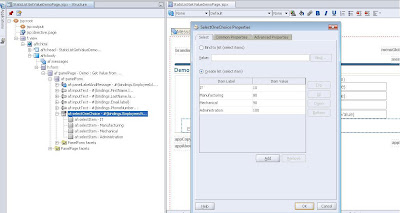
Following steps show how to get the value from this list at runtime.
In the page definition file following code gets added.
<list id="EmployeesView1DepartmentId" IterBinding="EmployeesView1Iterator" StaticList="false" ListOperMode="0" ListIter="DepartmentsViewIterator">
<AttrNames><Item Value="DepartmentId"/></AttrNames>
<ListAttrNames>
<Item Value="DepartmentId"/>
</ListAttrNames>
<ListDisplayAttrNames>
<Item Value="DepartmentName"/>
</ListDisplayAttrNames>
</list>
Following steps show how to get the value of a dynamic list at run time.
public void getDynamicListValue(ValueChangeEvent valueChangeEvent) {
// get the bindings to the current page components
BindingContainer bindings = getBindings();
try {
//get the control binding to the id of the List as written in the Page Definition file
JUCtrlListBinding listBinding = (JUCtrlListBinding)bindings.get("EmployeesView1DepartmentId");
// as this is a value change listener method, so the list binding contains the OLD value of the combo box and not the NEW one.
//The new value is available there in the valueChangeEvent as the index. So point the list binding to current selected index first
listBinding.setSelectedIndex(Integer.parseInt(valueChangeEvent.getNewValue().toString()));
// Now receive the selected row of the list binding
Row selectedValue = (Row) listBinding.getSelectedValue();
//from this row you can get the desired attributes
System.out.println( "Item Label :" + selectedValue.getAttribute("DepartmentName"));
System.out.println("Item Value :" + selectedValue.getAttribute("DepartmentId"));
}
catch (Exception ex)
{ex.printStackTrace();}
}
When you run the page and select an item from the combo box, the label and value of selected item are displayed in the Jdeveloper Console. Once you have got the values you can do whatever you want.
- Define the valueChangeListener Property of the DepartmentId field to some method of a bean. e.g. SelectOneChoiceValueGetter:getSelectOneChoiceValue(ValueChangeEvent valueChangeEvent)
- Now go to the above value change listener method and add the following code
public void getSelectOneChoiceValue(ValueChangeEvent valueChangeEvent) {
// take the selectOneChoice object from the valueChangeEvenet
CoreSelectOneChoice selectOneChoice = (CoreSelectOneChoice)valueChangeEvent.getSource();
//get the LOV items list object
List selectOnechoiceItemList = selectOneChoice.getChildren();
//traverse the list and get all the children items
for (int i = 0; i < selectOnechoiceItemList.size(); i++) {
if (selectOnechoiceItemList.get(i) instanceof CoreSelectItem) {
//get list item
CoreSelectItem csi = (CoreSelectItem)selectOnechoiceItemList.get(i);
// get the new value selected and type cast it into actual class
oracle.jbo.domain.Number num = (Number)valueChangeEvent.getNewValue();
//check if the list value is similar to the new selected value
if ((((String)csi.getValue()).equals(num.toString()))) {
System.out.println("Item Label :" + csi.getLabel());
System.out.println("Item Value :" + csi.getValue());
// here is your value. store it or print it
}
}
}
}
2. Dynamic List
Dynamic list is created when the number of values to be shown in the list box are not fixed. e.g. a new department can be added in an organisation in future and hence the newly added department is expected to appear in the list box at run time. In this case we create a dynamic list where the vales of the list box come at runtime from some master source. Following image shows the dynamic list created for the Department Id.
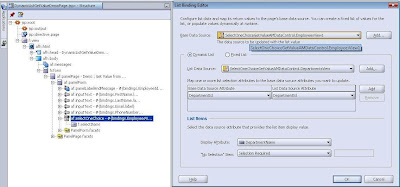
In the page definition file following code gets added.
<list id="EmployeesView1DepartmentId" IterBinding="EmployeesView1Iterator" StaticList="false" ListOperMode="0" ListIter="DepartmentsViewIterator">
<AttrNames><Item Value="DepartmentId"/></AttrNames>
<ListAttrNames>
<Item Value="DepartmentId"/>
</ListAttrNames>
<ListDisplayAttrNames>
<Item Value="DepartmentName"/>
</ListDisplayAttrNames>
</list>
Following steps show how to get the value of a dynamic list at run time.
- As defined earlier for static list, define the valueChangeListener Property of the DepartmentId field to some method of a bean. e.g. DynamicListValueGetter:getDynamicListValue(ValueChangeEvent valueChangeEvent)
- Now go to the above value change listener method and add the following code
public void getDynamicListValue(ValueChangeEvent valueChangeEvent) {
// get the bindings to the current page components
BindingContainer bindings = getBindings();
try {
//get the control binding to the id of the List as written in the Page Definition file
JUCtrlListBinding listBinding = (JUCtrlListBinding)bindings.get("EmployeesView1DepartmentId");
// as this is a value change listener method, so the list binding contains the OLD value of the combo box and not the NEW one.
//The new value is available there in the valueChangeEvent as the index. So point the list binding to current selected index first
listBinding.setSelectedIndex(Integer.parseInt(valueChangeEvent.getNewValue().toString()));
// Now receive the selected row of the list binding
Row selectedValue = (Row) listBinding.getSelectedValue();
//from this row you can get the desired attributes
System.out.println( "Item Label :" + selectedValue.getAttribute("DepartmentName"));
System.out.println("Item Value :" + selectedValue.getAttribute("DepartmentId"));
}
catch (Exception ex)
{ex.printStackTrace();}
}